class: center, middle, inverse, title-slide # Workshop 3: Introduction to ggplot2 ## QCBS R Workshop Series ### Québec Centre for Biodiversity Science --- ## Introduction - To follow along: Code and .HTML available at http://qcbs.ca/wiki/r/workshop3 - Recommendation: * create your own new script * refer to provided code only if needed * avoid copy pasting or running the code directly from script - ggplot2 is also hosted on Github: https://github.com/hadley/ggplot2 --- ## Outline 1. Introduction 2. ggplot mechanics 3. Importance of data structure 4. Basic plot 5. Aesthetic 6. Geom * point, line, Histogram, box plot * Error bar, sgnificant value & linear regression 7. Fine tunning * Color * Theme 8. Miscellaneous --- ## Introduction : Why use R for plotting? <div style="text-align:center"> <img src="images/Divided_reproducible workflow.png" height=85% width=85%> </div> --- ## Introduction : Why use R for plotting? <div style="text-align:center"> <img src="images/Divided_reproducible workflow2.png" height=85% width=85%> </div> --- ## Introduction : Why use R for plotting? Beautiful and flexible graphics! <img src="workshop03-en_files/figure-html/unnamed-chunk-3-1.png" width="504" style="display: block; margin: auto;" /> --- ## Introduction - Have you created plots? * What kind of plot? * Which software? - Have you plotted in R? * `base` R, `lattice`? * `ggplot2`? --- class: inverse, center, middle # ggplot2 --- ## ggplot2 The `ggplot2` package lets you make beautiful and customizable plots of your data. It implements the grammar of graphics, an easy to use system for building plots. <div style="text-align:center"> <img src="images/ggplot2_logo.png" height=40% width=40%> </div> --- ## Introduction Required packages ```r install.packages("ggplot2") library(ggplot2) ``` --- ## Grammar of Graphics (GG) A graphic is made of different layers: * aesthetics (`aes`) * transformation * geometries (`geoms`) * axis (coordinate system) * scales <img src="images/Layers_ggplot.png" height=100% width=100%> --- ```r ggplot(data = iris, # Data aes(x = Sepal.Length, # Your X-value y = Sepal.Width, # Your Y-value col = Species)) + # Aesthetics geom_point() + # Geometry geom_smooth(method = "lm") + # Linear regression ggtitle("My fabulous graph")+ # Title theme(plot.title= element_text(color="red", #| size=14, #| Theme face="bold.italic")) #| ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-5-1.png" width="360" style="display: block; margin: auto;" /> --- ## Importance in data structure ```r head(iris[,c("Sepal.Length","Sepal.Width","Species")], n = 5) # Sepal.Length Sepal.Width Species # 1 5.1 3.5 setosa # 2 4.9 3.0 setosa # 3 4.7 3.2 setosa # 4 4.6 3.1 setosa # 5 5.0 3.6 setosa ``` `ggplot(data = iris, aes(`**x = Sepal.Length**`,` **y = Sepal.Width**`, `**col = Species**`))` --- ## A simple Example with iris database .small[ .pull-left[ **Inheritance from ggplot** ```r ggplot(data = iris, # Data aes(x = Sepal.Length, # Your X-value y = Sepal.Width)) + # Your Y-value geom_point() # Geometry ``` **Storing ggplot in object** ```r p <- ggplot(data = iris, aes(x = Sepal.Length, y = Sepal.Width)) q <- p + geom_point() q # Print your final plot ``` ] .pull-right[ **No inheritance from ggplot** ```r ggplot() + geom_point(data = iris, aes(x = Sepal.Length, y = Sepal.Width)) ``` **Adding layer from ggplot object** ```r s <- ggplot() s <- s + geom_point(data = iris, aes(x = Sepal.Length, y = Sepal.Width)) s ``` ] ] --- ## GGplot dynamics: base layer ```r ggplot() + scale_x_continuous() + scale_y_continuous() ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-11-1.png" width="360" style="display: block; margin: auto;" /> --- ## GGplot dynamics: Data layer ```r ggplot(data = iris, aes(x = Sepal.Length, y = Sepal.Width)) + xlab("x = Sepal Lenght") + ylab("y = Sepal Width") ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-12-1.png" width="360" style="display: block; margin: auto;" /> --- ## GGplot dynamics: Gemoetric Layer ```r ggplot(data = iris, aes(x = Sepal.Length, y = Sepal.Width)) + xlab("x = Sepal Lenght") + ylab("y = Sepal Width") + geom_point() ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-13-1.png" width="360" style="display: block; margin: auto;" /> --- ## Challenge #1 ![:cube]() * Draw your 1rst (gg)plot: data | geom | x value | y value :-------------:|:-------------:|:-------------:|:-------------: iris|geom_point|Petal length|Petal width --- ## Solution Challenge 1# ![:cube]() ```r ggplot(data = iris, aes(x = Petal.Length, y = Petal.Width)) + geom_point() ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-14-1.png" width="360" style="display: block; margin: auto;" /> --- ## Saving plots in RStudio <div style="text-align:center"> <img src="images/save_image.png" height=80% width=80%> </div> --- ## Saving plots in code `ggsave()` will write directly to your working directory all in one line of code and you can specify the name of the file and the dimensions of the plot: .small[ ```r my1rstPlot <- ggplot(data = iris, aes(x = Petal.Length, y = Petal.Width)) + geom_point() ggsave("my1rstPlot.pdf", my1rstPlot, height = 8.5, width = 11, units = "in") ``` ] .comment[Note that vector format (e.g., pdf, svg) are often preferable choice compared to raster format (jpeg, png, ...)] .comment[Other methods to save image `?pdf` `?jpeg`] --- ## Aesthetic Different aesthetic are available to help you distinguish different class, group & structure within your data: <img src="workshop03-en_files/figure-html/unnamed-chunk-16-1.png" width="576" style="display: block; margin: auto;" /> --- ## Example of aesthetic: color .small[ .pull-left[ ```r #Without Color ggplot(data = iris, aes(x = Sepal.Length, y = Sepal.Width)) + geom_point() ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-17-1.png" width="432" style="display: block; margin: auto;" /> ] .pull-right[ ```r ggplot(data = iris, aes(x = Sepal.Length, y = Sepal.Width, color = Species)) + geom_point() + theme(legend.position = 'bottom') ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-18-1.png" width="432" style="display: block; margin: auto;" /> ] ] --- ## Challenge #2 ![:cube]() Produce a colourful plot from built in data such as the `Iris`, `CO2` or the `msleep` dataset <br> Data|x value|y value| Aesthetic :-------------:|:-------------:|:-------------:|:-------------: iris|Petal.Length|Petal.Width|Species CO2|conc|uptake|Treatment & Type msleep|log10(bodywt)|awake| vore & conservation --- ## Solution to challenge #2 ![:cube]() Example using `iris` database ```r data(iris) iris.plot <- ggplot(data = iris, aes(x = Petal.Length, y = Petal.Width, color = Species)) + geom_point() iris.plot ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-19-1.png" width="432" style="display: block; margin: auto;" /> --- ## Solution to challenge #2 ![:cube]() Example using `CO2` database ```r data(CO2) CO2.plot <- ggplot(data = CO2, aes(x = conc, y = uptake, colour = Treatment)) + geom_point() CO2.plot ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-20-1.png" width="432" style="display: block; margin: auto;" /> --- ## Solution to challenge #2 ![:cube]() Example using `msleep` database ```r data(msleep) msleep.plot <- ggplot(data = msleep, aes(x = log10(bodywt), y = awake, colour = vore, shape= conservation)) + geom_point() msleep.plot ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-21-1.png" width="432" style="display: block; margin: auto;" /> --- ## Facet: `Iris` ```r ggplot(data = iris, aes(x = Sepal.Length, y = Sepal.Width, color = Species)) + geom_point() + facet_grid(~Species, scales = "free") ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-22-1.png" width="504" style="display: block; margin: auto;" /> --- ## Facet: `CO2` <img src="workshop03-en_files/figure-html/unnamed-chunk-23-1.png" width="504" style="display: block; margin: auto;" /> --- ## Fine tuning - colours Colour: Manual_colour .small[ ```r iris.plot <- ggplot(data = iris, aes(x = Sepal.Length, y = Sepal.Width, color = Species)) + geom_point() iris.plot + scale_colour_manual(values = c("setosa" = "red", "versicolor" = "darkgreen", "virginica"="blue")) ``` ] .pull-left[ **Classic** <img src="workshop03-en_files/figure-html/unnamed-chunk-25-1.png" width="432" style="display: block; margin: auto;" /> ] .pull-right[ **Manual** <img src="workshop03-en_files/figure-html/unnamed-chunk-26-1.png" width="432" style="display: block; margin: auto;" /> ] --- ## Fine tuning - gradient colours for quantitative variable *quantitavie variable: `Petal.Length`* ```r iris.plot.petal <- ggplot(data=iris, aes(..., color = Petal.Length)) ``` .pull-left[ <img src="workshop03-en_files/figure-html/unnamed-chunk-28-1.png" width="432" style="display: block; margin: auto;" /> ] .pull-right[ <img src="workshop03-en_files/figure-html/unnamed-chunk-29-1.png" width="432" style="display: block; margin: auto;" /> ] --- ## Fine tuning - colours Coulours theme ```r install.packages("RColorBrewer") require(RColorBrewer) ``` <div style="text-align:center"> <img src="images/Rcolour Brewers palette prez.png" height=100% width=100%> </div> --- ## Fine tuning - colours Coulours theme: RColorBrewer ```r iris.plot + scale_color_brewer(palette = "Dark2") ``` .pull-left[ **Classic** <img src="workshop03-en_files/figure-html/unnamed-chunk-32-1.png" width="432" style="display: block; margin: auto;" /> ] .pull-right[ **Dark2** <img src="workshop03-en_files/figure-html/unnamed-chunk-33-1.png" width="432" style="display: block; margin: auto;" /> ] --- ## Fine tuning - colours Blind color friendly: presentation purpose ```r cbbPalette <- c("#000000", "#E69F00", "#56B4E9", "#009E73", "#F0E442", "#0072B2", "#D55E00", "#CC79A7") iris.plot + scale_color_manual(values=cbbPalette) ``` .pull-left[ **Classic** <img src="workshop03-en_files/figure-html/unnamed-chunk-35-1.png" width="432" style="display: block; margin: auto;" /> ] .pull-right[ **Blind color friendly** <img src="workshop03-en_files/figure-html/unnamed-chunk-36-1.png" width="432" style="display: block; margin: auto;" /> ] --- ## Fine tuning - colours black & white: grey scale for publication purpose ```r iris.plot + scale_color_grey() ``` .pull-left[ **Defaut** <img src="workshop03-en_files/figure-html/unnamed-chunk-38-1.png" width="432" style="display: block; margin: auto;" /> ] .pull-right[ **Grey scale** <img src="workshop03-en_files/figure-html/unnamed-chunk-39-1.png" width="432" style="display: block; margin: auto;" /> ] --- ## Fine tuning - theme Theme: classic for publication purpose ```r iris.plot + scale_color_grey() + theme_classic() ``` .pull-left[ **Defaut** <img src="workshop03-en_files/figure-html/unnamed-chunk-41-1.png" width="432" style="display: block; margin: auto;" /> ] .pull-right[ **Classic** <img src="workshop03-en_files/figure-html/unnamed-chunk-42-1.png" width="432" style="display: block; margin: auto;" /> ] --- ## Challenge #3 ![:cube]() Explore a new `geom()` and other plot elements with your own data or built in data ```r data(msleep) data(OrchardSprays) ``` --- ## Solution challenge #3 ![:cube]() ```r box.plot <- ggplot(data = OrchardSprays, aes(x = treatment, y = decrease)) + geom_boxplot() box.plot ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-44-1.png" width="360" style="display: block; margin: auto;" /> --- ## Geom: GGplot <div style="text-align:center"> <img src="images/plots_in_various_contexts.png" height=85% width=85%> </div> --- ## Base plot <div style="text-align:center"> <img src="images/plots_in_various_contexts_baseplot.png" height=85% width=85%> </div> --- ## Blank plot .small[ .pull-left[ *GGplot2* ```r ggplot(mtcars, aes(wt, mpg)) + geom_blank() ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-45-1.png" width="360" style="display: block; margin: auto;" /> ] .pull-right[ *Base Plot* ```r plot(mpg~wt, data = mtcars, type = "n") ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-46-1.png" width="360" style="display: block; margin: auto;" /> ]] --- ## Histogram .small[ .pull-left[ *GGplot2* ```r qplot(rnorm(100)) + geom_histogram() ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-47-1.png" width="360" style="display: block; margin: auto;" /> ] .pull-right[ *Base Plot* ```r hist(rnorm(100)) ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-48-1.png" width="360" style="display: block; margin: auto;" /> ]] --- ## Barplot: value significance .small[ .pull-left[ *GGplot2* ```r qplot(rnorm(100)) + geom_histogram() ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-49-1.png" width="360" style="display: block; margin: auto;" /> ] .pull-right[ *Base Plot* ```r counts <- table(mtcars$gear) barplot(counts, main="Car Distribution", xlab="Number of Gears", border=NA) ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-50-1.png" width="360" style="display: block; margin: auto;" /> ]] --- ## Plot: linear regression --- ## Plot with categories --- ## Boxplot with significant value only --- ## Violoin Plot --- ## Plot with errors bars --- ## Map --- ## Density Graph --- ## Dendogram --- ## Ordination: PCA --- ## Geom_point & Geom_line .alert[put ggplot vs base plot] ```r CO2.plot.facet.baline <- CO2.plot.facet + geom_line() CO2.plot.facet.line <- CO2.plot.facet + geom_line(aes(group=Plant)) multiplot(CO2.plot.facet.baline, CO2.plot.facet.line) ``` <img src="workshop03-en_files/figure-html/unnamed-chunk-51-1.png" width="360" style="display: block; margin: auto;" /> --- class: inverse, center, middle # Miscellaneous --- ## Miscellaneous * .alert[Put other Crazy R visualisation] * .alert[A time for a tour on r-graph-gallery.com] * .alert[Itroduce Dynamics representative] * .alert[May be let the choice to the current speaker to show its own tricks and/or own data visu] --- # Miscellaneous : `qplot()` vs `ggplot()` ```r qplot(data = iris, x = Sepal.Length, xlab = "Sepal Length (mm)", y = Sepal.Width, ylab = "Sepal Width (mm)", main = "Sepal dimensions") ggplot(data = iris, aes(x = Sepal.Length, y = Sepal.Width)) + geom_point() + xlab("Sepal Length (mm)") + ylab("Sepal Width (mm)") + ggtitle("Sepal dimensions") ``` --- ## Available elements [Data Visualization with ggplot2 Cheat Sheet](https://www.rstudio.com/wp-content/uploads/2015/03/ggplot2-cheatsheet.pdf) <div style="text-align:center"> <img src="images/available_geom.png" height=50% width=50%> </div> --- ## Additional resources `help(package = ggplot)` http://ggplot2.tidyverse.org/reference/ <div> <img src="images/ggplot_book.jpg"/> <img src="images/grammar_of_graphics.jpg"/> </div> --- class: inverse, center, bottom # Merci d'avoir participé! 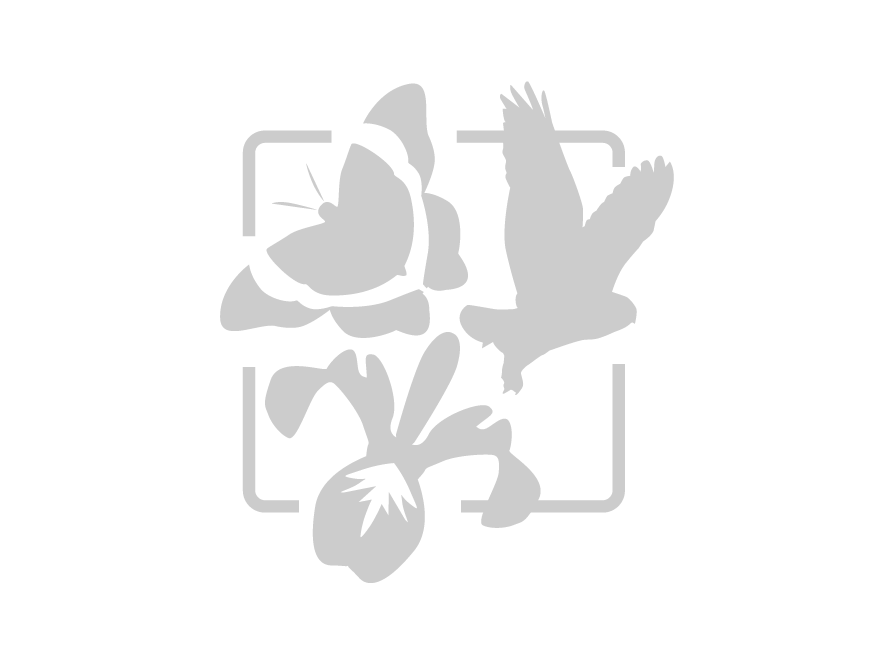